Learning how to write to files in Deno is an indispensable skill for developers aiming to fully leverage this cutting-edge JavaScript and TypeScript runtime. Whether you're constructing server-side applications, developing automation scripts, or managing data processing tasks, understanding how to effectively write to files in Deno will substantially elevate your development capabilities. This guide will provide an in-depth walkthrough of writing to files in Deno, covering foundational concepts to advanced techniques.
In today's rapidly evolving digital landscape, proficiency in file operations is essential for developers. Deno stands out with its secure and modular architecture, offering a robust framework for handling file operations, including writing to files. This article delves into Deno's file-writing capabilities, ensuring that developers can fully exploit its potential to enhance their projects.
By the conclusion of this guide, you'll have a thorough understanding of how to write to files in Deno, complete with practical examples, best practices, and strategies to avoid common pitfalls. Let's embark on this journey to explore the world of Deno file operations.
Read also:Access Iot Device Behind Nat
Table of Contents:
- Understanding Deno
- Fundamentals of Writing Files in Deno
- Synchronous File Writing
- Asynchronous File Writing
- Managing File Encoding
- Appending to Files
- Advanced Techniques
- Common Errors and Troubleshooting
- Performance Considerations
- Best Practices for File Writing
Understanding Deno
Deno is a contemporary runtime for JavaScript and TypeScript designed to deliver a secure and efficient environment for executing code outside the browser. Created by Ryan Dahl, the originator of Node.js, Deno addresses several limitations and concerns associated with Node.js. One of its standout features is its inherent support for secure and efficient file operations.
Writing to files in Deno is a critical operation enabling developers to persist data, create logs, and manage configuration files. Deno's approach to file operations prioritizes security and ease of use, making it an exceptional choice for developers who value both functionality and safety.
Why Select Deno for File Operations?
- Secure by default with permission-based access control: Deno ensures that file operations are conducted securely by requiring explicit permissions.
- Support for synchronous and asynchronous file operations: Deno accommodates both synchronous and asynchronous methods, offering flexibility based on the needs of your application.
- First-class support for TypeScript: Deno enables type-safe development, making it easier to write and maintain complex applications.
- Modern syntax aligned with the latest JavaScript standards: Deno incorporates the latest JavaScript features, ensuring your code remains modern and efficient.
Fundamentals of Writing Files in Deno
Grasping the basics of writing to files in Deno is the initial step toward mastering this vital operation. Deno provides an intuitive API for file manipulation, enabling developers to write data to files effortlessly. By utilizing Deno's straightforward methods, you can handle various data types and file formats with ease.
Writing to a file in Deno can be achieved using the writeTextFile
or writeFile
methods, depending on your specific requirements. These methods offer versatility in managing different data types and file formats, ensuring flexibility in your file-writing operations.
Basic Syntax for Writing to Files
Here's a basic example of writing text to a file using Deno:
Read also:Jeff Carriveau
javascript Deno.writeTextFile("example.txt", "Hello, Deno!");
This code snippet creates a file named "example.txt" and writes the string "Hello, Deno!" to it. If the file already exists, its contents will be overwritten, demonstrating the simplicity and power of Deno's file-writing capabilities.
Synchronous File Writing
Synchronous file writing in Deno is particularly advantageous for scenarios where you need to confirm that file operations are completed before proceeding to subsequent tasks. Although synchronous operations may block the event loop, they are ideal for small scripts or one-off tasks where simplicity is crucial.
Utilizing the writeTextFile
method in a synchronous manner is straightforward:
javascript Deno.writeTextFileSync("example.txt", "Synchronous write example.");
This code writes the specified text to the file "example.txt" without requiring any callbacks or promises. It simplifies the process of writing to files in scenarios where blocking the event loop is not a concern.
Advantages of Synchronous Writing
- Simplified code structure for small scripts: Synchronous writing results in cleaner and more straightforward code for simple applications.
- Easier debugging due to linear execution flow: Debugging synchronous code is generally more straightforward since the code executes in a predictable order.
Asynchronous File Writing
For more complex applications, asynchronous file writing is preferred to prevent blocking the event loop. Deno provides robust asynchronous methods that allow developers to handle file operations efficiently without compromising performance. By adopting asynchronous operations, your application can maintain responsiveness and scalability.
To write to a file asynchronously, you can use the writeTextFile
method with promises:
javascript await Deno.writeTextFile("example.txt", "Asynchronous write example.");
This approach ensures that other tasks can continue running while the file-writing operation is in progress. It is especially beneficial for applications that require handling multiple file operations concurrently, ensuring optimal performance and resource utilization.
Benefits of Asynchronous Writing
- Enhanced application responsiveness and scalability: Asynchronous operations prevent the application from being blocked, allowing it to handle multiple tasks simultaneously.
- Efficient handling of multiple file operations: Asynchronous methods enable developers to manage numerous file operations concurrently, improving overall application performance.
Managing File Encoding
File encoding plays a crucial role when writing to files in Deno. By default, Deno employs UTF-8 encoding, which is suitable for most text-based operations. However, in cases where you need to work with alternative encodings, Deno provides the flexibility to specify the encoding explicitly.
To write to a file with a specific encoding, you can use the writeFile
method and pass the appropriate encoding as an option:
javascript await Deno.writeFile("example.bin", new Uint8Array([65, 66, 67]), { mode: 0o666 });
This example demonstrates writing binary data to a file with specified permissions. By specifying the encoding and permissions explicitly, you gain greater control over the file-writing process, ensuring compatibility and security.
Choosing the Right Encoding
- UTF-8 for standard text files: UTF-8 is widely used for text files due to its compatibility with most systems and applications.
- Binary encoding for handling raw data: Binary encoding is essential for managing raw data, such as images or executables, ensuring the integrity of the data.
- Custom encodings for specialized use cases: In scenarios requiring unique encodings, Deno's flexibility allows developers to tailor the file-writing process to their specific needs.
Appending to Files
There are situations where instead of overwriting a file, you may need to append data to an existing file. Deno simplifies this process by providing the open
method with the append flag, enabling seamless appending of data.
Here's how you can append text to a file:
javascript const file = await Deno.open("example.txt", { write: true, create: true, append: true }); await Deno.writeText(file.rid, "Appending to the file."); await file.close();
This code opens the file in append mode and adds the specified text to the end of the file. By leveraging Deno's append functionality, you can efficiently manage incremental data additions without overwriting existing content.
Best Practices for Appending
- Always close the file after completing the operation: Ensuring files are properly closed prevents data corruption and resource leaks.
- Implement error handling to manage potential issues: Incorporating robust error handling ensures that your application can gracefully handle unexpected issues during file operations.
Advanced Techniques
For developers seeking to maximize Deno's file-writing capabilities, advanced techniques can unlock even greater potential. These techniques encompass working with streams, managing large files, and optimizing performance for high-throughput applications. By adopting advanced methods, you can enhance the efficiency and scalability of your file-writing operations.
Using Streams for Efficient File Operations
Streams are a powerful feature in Deno that facilitate efficient handling of large data sets. By utilizing streams, you can process data in smaller chunks, reducing memory usage and improving performance. This approach is especially beneficial for applications that require handling large files or data streams.
javascript const file = await Deno.open("largefile.txt", { write: true, create: true }); const writer = file.writable; const encoder = new TextEncoder(); await writer.write(encoder.encode("Large file content.")); await file.close();
Common Errors and Troubleshooting
Although Deno provides a robust framework for file operations, developers may encounter errors during development. Understanding common issues and how to troubleshoot them is essential for maintaining smooth operations and ensuring the reliability of your applications.
Permission Denied Errors
One prevalent issue is encountering permission denied errors when attempting to write to files. To resolve this, ensure that you have the necessary permissions by running Deno with the appropriate flags:
bash deno run --allow-write your_script.ts
Performance Considerations
Optimizing performance is critical for applications involving frequent file operations. Deno offers several strategies to enhance performance, such as leveraging asynchronous operations, utilizing streams, and minimizing file I/O overhead. By implementing these strategies, you can improve the efficiency and responsiveness of your applications.
Tips for Improving Performance
- Use asynchronous methods for non-blocking operations: Asynchronous methods prevent the application from being blocked, ensuring smooth execution of concurrent tasks.
- Batch write operations to reduce I/O calls: Batching write operations minimizes the number of I/O calls, enhancing overall performance.
- Utilize caching mechanisms where applicable: Caching frequently accessed data can significantly reduce the need for repetitive file operations, improving performance.
Best Practices for File Writing
Adopting best practices can significantly enhance the reliability and maintainability of your Deno-based applications. Here are some key practices to consider when writing to files in Deno:
- Always validate input data before writing to files: Validating input data ensures data integrity and prevents potential security vulnerabilities.
- Implement error handling to manage unexpected issues gracefully: Robust error handling mechanisms enable your application to handle unforeseen issues without compromising functionality.
- Follow security guidelines to protect sensitive data: Adhering to security best practices safeguards sensitive data, ensuring the confidentiality and integrity of your files.
By following these best practices, you can ensure that your file-writing operations in Deno are both efficient and secure, enhancing the overall quality of your applications.
Conclusion
In summary, mastering the skill of writing to files in Deno is invaluable for developers. From fundamental operations to advanced techniques, Deno provides a
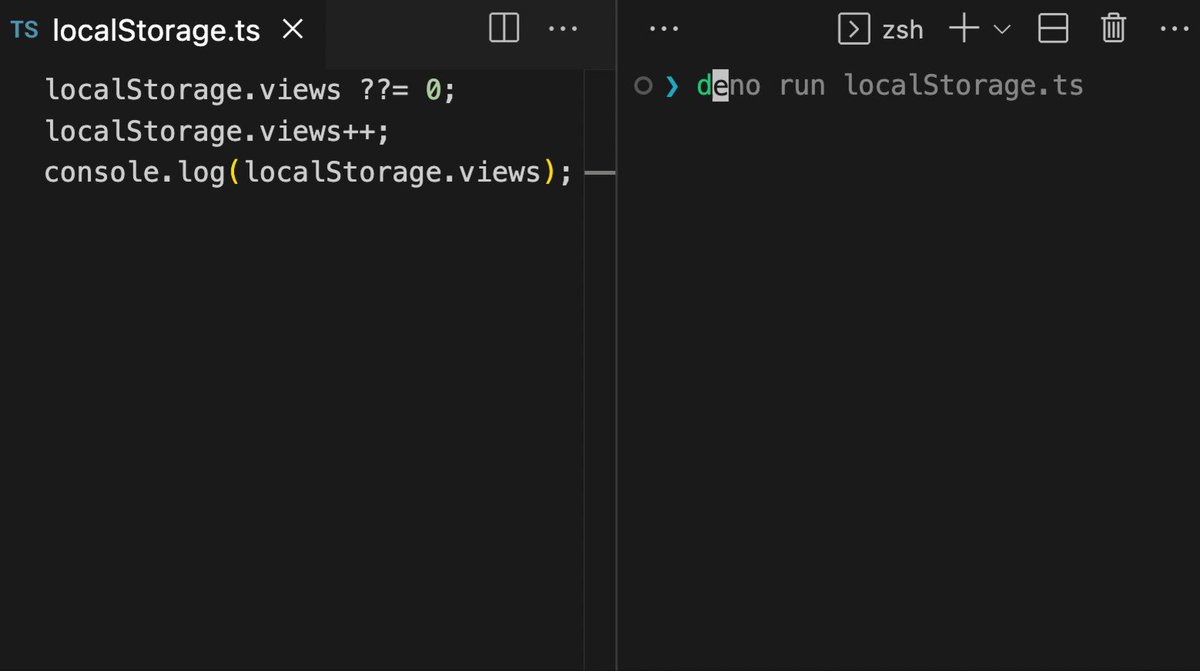
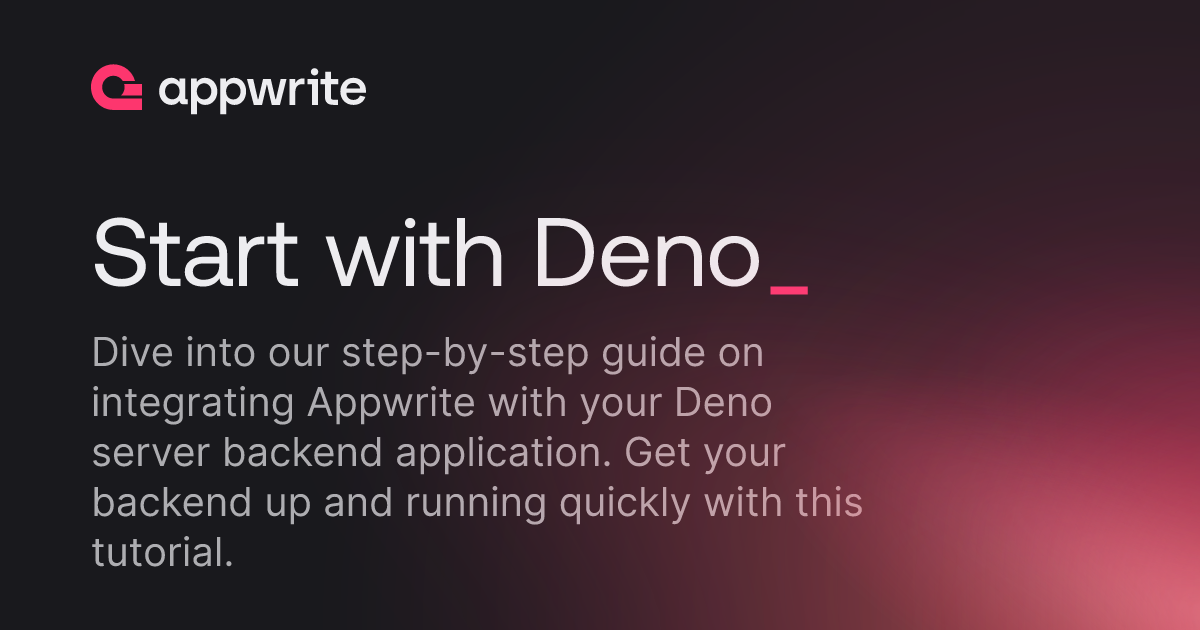